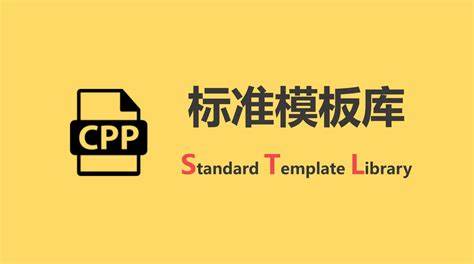
C++篇-STL
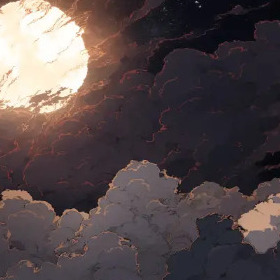
Preface
When it comes to data structures and algorithms,C++’s standard template library can greatly help you speed up your efficiency. However, the standard template library is a very large library, which contains many encapsulated data structure templates and algorithm implementations, so it is actually a bit difficult to memorize completely, and it is almost difficult to implement, which may be an NP problem. Yes, it seems as if our memories are always limited. Therefore, a good memory is not as good as the memory of a pen, and recording this learning process is also a process of continuous memory.
Guide
About STL
Something about C++ stl
STL encompasses the following core concepts
Imitation functions
Algorithm
Iterator
Space Configurator
Containers
Adapters
Learning priorities are given here:
- Containers
- Algorithm
- Iterator
- Adapters
- Space Configurator
- Imitation functions
note that this does not necessarily apply to all developers who are learning advanced features of C++. It’s a good habit to study selectively according to your needs.
Containers
collection of data structures
Before starting learning containers, you need to add some pre-knowledge such as the use of std::pair, and at the same time, it will also add some extended knowledge in the continuous recording process.
std::pair
1 | template<class T1,class T2> struct pair{} |
std::map
1 | //create a map with two pairs |
Summary
Reference
- Title: C++篇-STL
- Author: wensboy
- Created at : 2024-11-28 06:22:59
- Updated at : 2024-11-28 06:22:59
- Link: https://www.wensboy.site/2024/11/28/cplusplus-STL/
- License: This work is licensed under CC BY-NC-SA 4.0.